29. August 2021
Using LocalStack as Your AWS in Local Go Development
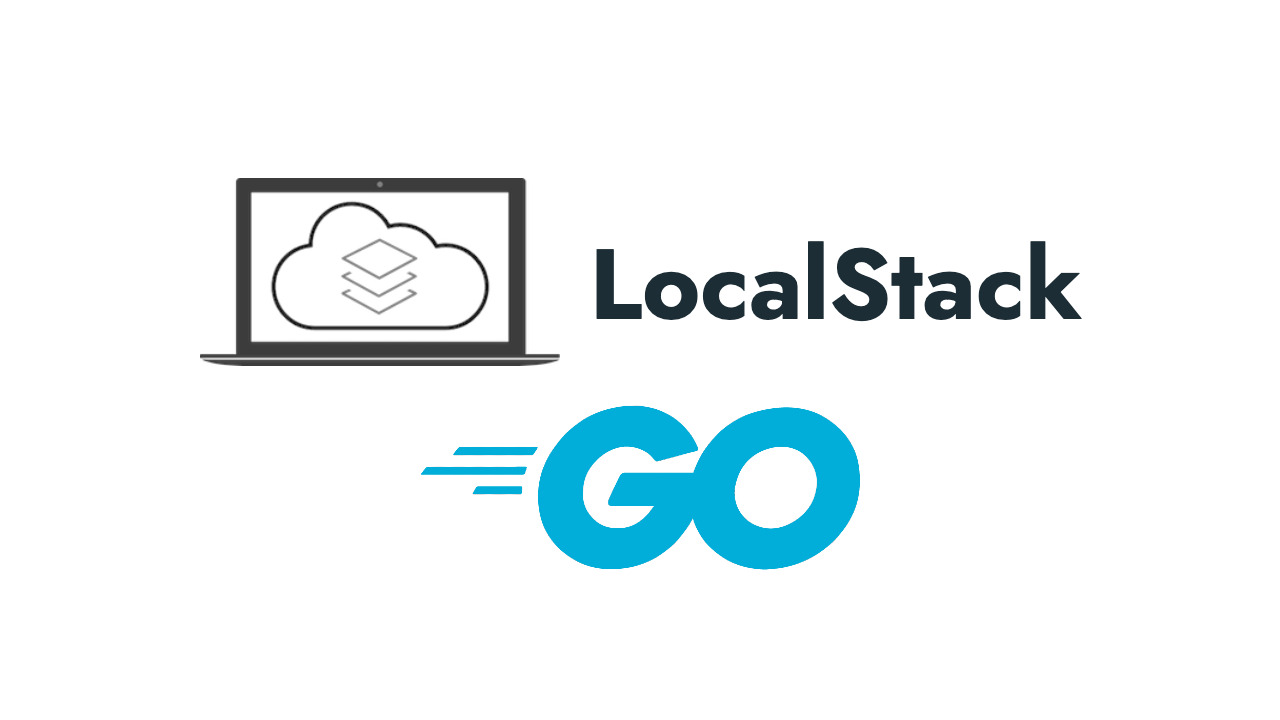
Have you ever think about having AWS in your local machine? Yes, you can. In this article, I will show you how to integrate your Go app with LocalStack.
For connecting your Go app to LocalStack, you need to have these things:
- Go app
- LocalStack
- AWS CLI
We will pick 2 AWS services, SNS and SQS. We will connect SNS and SQS, so if we publish a message to SNS topic, we can consume that message through SQS.
Setting up LocalStack
According to their Github Repo, LocalStack is a fully functional local AWS cloud stack. You can take a look in the Overview section, there are many AWS products supported.
To running LocalStack in your local, you can run using 3 methods using Pip, Docker, and Docker Compose. But, I recommend you to use Docker Compose. Because you can easily set the Environment Variable in docker-compose.yml
.
Download the docker-compose.yml
file from the original repository. If you have a wget
command, you can use this command to download the file.
|
|
Then, run the docker-compose file.
|
|
Note !!
If you face an error while running the docker-compose script, please downgrade the docker image version. As per this article release, I use 0.12.7 as the latest image version.
If your docker log like this, then it means your LocalStack is ready to use in localhost:4566
(default port)
AWS CLI
To interact with LocalStack, you need to have a AWS CLI. We will use the latest version, which is version 2. Please follow this installation guide.
If you are using MacOS, you can also install with Homebrew command.
brew install awscli
To check whether the installation is a success or not. Try to run aws --version
, it will print the version of AWS CLI
The AWS CLI docs can be found here : https://awscli.amazonaws.com/v2/documentation/api/latest/reference/index.html
Connecting AWS CLI to LocalStack
To connecting to LocalStack, everytime to execute the command in AWS CLie just add the argument --endpoint-url <your_localstack_url>
in the last. For example : aws sqs list-queues --endpoint-url http://localhost:4566
.
To make this process more simple, if you are using Linux or macOS just add this script to your ~/.bash_profile
or ~/.zshrc
file.
|
|
Then run, source ~/.bash_profile
or source ~/.zshrc
to reload that file.
So, every time you execute the command, you can ignore the --endpoint-url
args.
Before we proceed to SQS and SNS section. I already make the example code written in Golang using aws-sdk-go and aws-sdk-go-v2. You can clone the code to your local : http://github.com/vinbyte/aws-go-localstack-example
The SQS
Now, your LocalStack and AWS CLI is ready. It’s time to create your SQS Queue in LocalStack via AWS CLI. Let’s say your queue name is test-queue0
. So, the command is
|
|
After that, you will receive the SQS queue URL. Copy and paste it into your text editor. We gonna use it later.
Ok now we have our queue, next we will try this queue with our example Golang code. Navigate to /aws-sdk-go-v1/sqs/receive-message
folder or /aws-sdk-go-v2/sqs/receive-message
. Now, try to run :
|
|
The code will find the queue URL and listen for the incoming queue message.
Let’s try to send the queue message.
|
|
Then, you will see the MessageId
Also, in the Golang code, you will receive the message.
Ok, we successfully send and receive messages using SQS LocalStack. But, what about if you want to subscribe to the SNS topic? Is it possible to do? Yes, it is possible.
Setup The SNS
Before we send the message to SNS topic, we must create the topic first. Let’s create the topic name test-topic0
|
|
Then, we will receive the topic ARN. Again, keep it save in your text editor.
Nice, we have our SNS topic. We need to set the subscription to the SQS queue that we have before. But, if you take a look in AWS CLI above in SNS subscribe section (https://awscli.amazonaws.com/v2/documentation/api/latest/reference/sns/subscribe.html) we need to find our SQS ARN first. Remember your queue URL that I said to keep saved in your text editor? Yes, we will use it to find the queue attributes.
|
|
And we have the attributes, copy-paste the queue ARN in your text editor.
Back to SNS, we have all the ingredients to make the SNS subscription. Let’s make it.
|
|
Ok, now the queue test-queue0
is subscribed to test-topic0
. It means, that if we publish the message to the SNS topic, the SQS queue will consume it.
Lets try publish something using Golang. Run the SQS code like before we did.
|
|
Open the new terminal, navigate it to the /sns/publish-message/
folder. Feel free to choose your SDK version. Run the code and input the topic ARN along with your message.
|
|
After run that command, you will see the Message ID. It means, your message was successfully sent to the SNS topic and the SQS queue immediately consume it.
Alright, congratulations. You successfully having the AWS running in your local and make it work. If you want to try another AWS service, grab their example code here https://github.com/awsdocs/aws-doc-sdk-examples/tree/master/go or the v2 version here https://github.com/awsdocs/aws-doc-sdk-examples/tree/master/gov2.
See you guys. Bye-bye.